RAMP API¶
This is the full API documentation of the different RAMP packages.
RAMP database¶
ramp_database.model
: the database model¶
The ramp_database.model
defines the database structure which is used for
the RAMP events.
The database schema is summarized in the figure below:
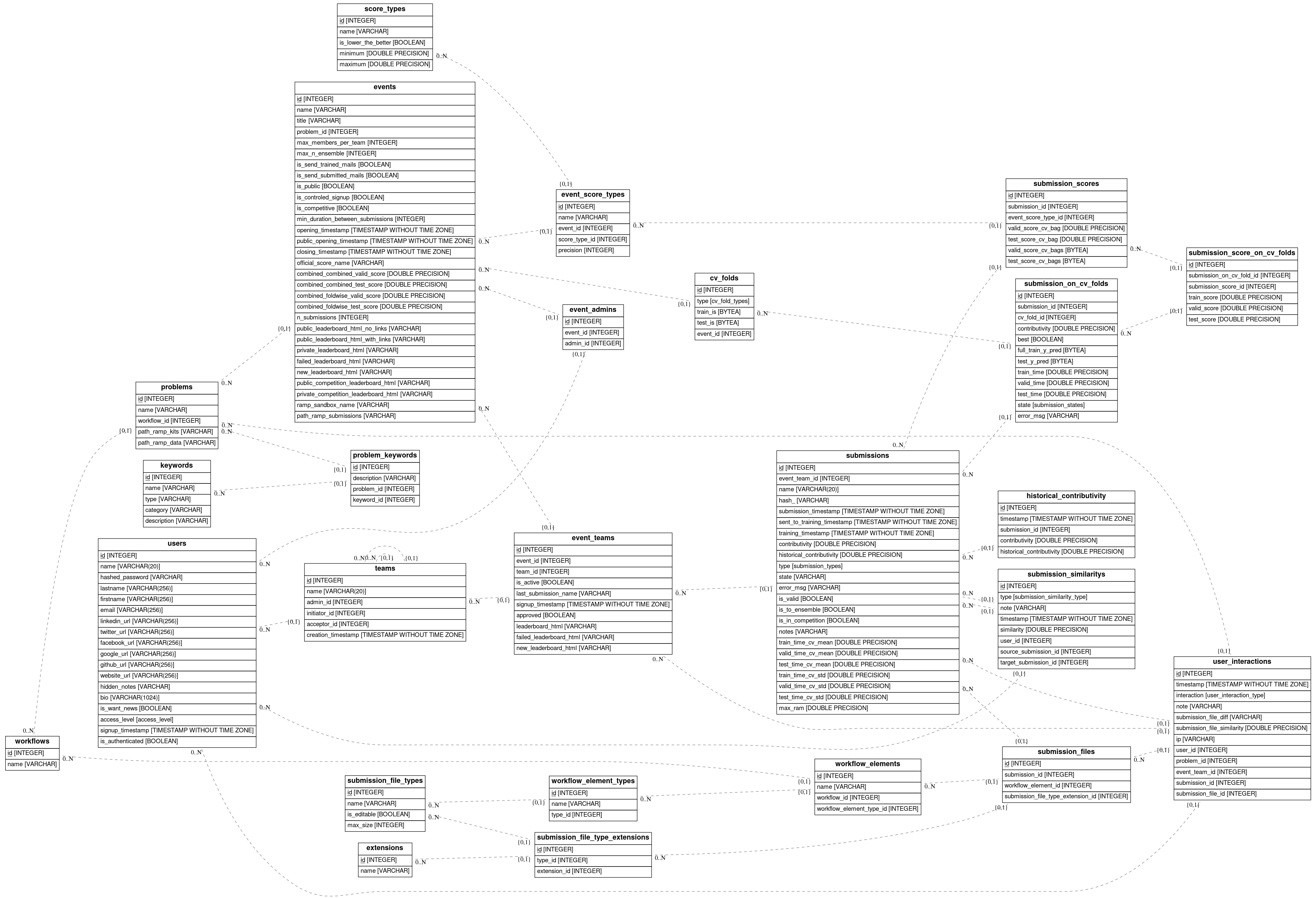
General tables¶
|
Extension table. |
|
Keyword table. |
|
UserInteraction table. |
Relationship tables¶
|
EventTeam table. |
|
EventAdmin table. |
|
EventScoreType table. |
|
ProblemKeyword table. |
|
SubmissionFileTypeExtension table. |
|
WorkflowElement table. |
|
WorkflowElementType table. |
ramp_database.tools
: the tools to communicate with the database¶
The ramp_database.tools
provides some routine to get and set
information from and in the database.
ramp_database.exceptions
: type of errors raise by the database¶
The ramp_database.exceptions
module include all custom warnings and
errors used in ramp-database
.
Error to raise when a submission is already present in the database. |
|
Error to raise when the merging of teams is failing. |
|
Error to raise when the file submitted is not present in the supposed location. |
|
Error to raise when the extension is not registered in the database. |
|
Error to raise when there is a duplicate in submission name. |
|
Error to raise when a submission was submitted to early. |
|
Error to raise when the state of the submission is unknown. |
ramp_database.testing
: functionalities to test database model and tools¶
The ramp_database.testing
module create facility functions to test the
tools and model of ramp-database
.
|
Add events in the database. |
|
Add dummy users in the database. |
|
Add dummy problems into the database. |
|
Create an empty test database and the setup the files for RAMP. |
|
Create a toy dataset with couple of users, problems, events. |
|
Only setup the database by adding some data. |
|
Clone ramp-kit and ramp-data repository and setup it up. |
|
Setup the files’ extensions and types. |
|
Sign up user to the events in the database. |
|
Submit all starting kits. |
ramp_database.utils
: setup and connect RAMP database¶
The ramp_database.utils
module provides tools to setup and connect to
the RAMP database.
|
Check if a password is the same than the hashed password. |
|
Hash a password. |
|
Create a sqlalchemy engine and session to interact with the database. |
|
Connect to a database and provide a session to make some operation. |
RAMP engine¶
The RAMP engine is made of a dispatcher to orchestrate the training and evaluation of submissions which are processed using workers. Since there is a dispatcher for each RAMP event, we provide a daemon which will start dispatcher for each open event.
RAMP Dispatcher¶
|
RAMP daemon starting dispatchers for open challenges. |
|
Dispatcher which schedule workers and communicate with the database. |
RAMP Workers¶
|
Metaclass used to build a RAMP worker. |
|
Local worker which uses conda environment to dispatch submission. |
|
Dask distributed worker |
|
Run RAMP submissions on Amazon. |
RAMP frontend¶
The RAMP frontend is the development of the website. It uses extensively Flask.
|
Create the RAMP Flask app and register the views. |
ramp_frontend.views
: views used on the frontend to interact with the user¶
The ramp_frontend.views
defines all operations a user can need to deal
with the RAMP backend.
General views¶
Default landing page. |
|
RAMP description request. |
|
Page related to RAMP offers for teaching classes. |
|
Page reviewing problems organized by ML themes. |
|
|
Page which give details about a keyword. |
Authentication views¶
Admin views¶
Approve new user to log-in and sign-up to events. |
|
|
Approve a single user. |
|
Approve a user for a specific event. |
|
Update the parameters of an event. |
Show the user interactions recorded on the website. |
|
|
Show information about all submissions for a given event. |
Leaderboard views¶
|
Landing page of all user’s submission information. |
|
Landing page showing all user’s submissions information. |
|
Landing page for the competition leaderboard for all users. |
|
Landing page for the private leaderboard. |
Landing page for the private competition leaderboard. |
Submission views¶
Landing page showing all the RAMP problems. |
|
|
Landing page for a single RAMP problem. |
|
Landing page for a given event. |
|
Landing page to sign-up to a specific RAMP event. |
|
Landing page for the user’s sandbox. |
|
|
|
The landing page to credit other submission when a user submit is own. |
|
Landing page of the plot illustrating the score evolution over time for a specific RAMP event. |
|
Rendering submission codes using templates/submission.html. |
|
Rendering submission codes using templates/submission.html. |
Utilities¶
|
Redirect the page to the credit landing page. |
|
Redirect the page to the sandbox landing page. |
|
Redirect the page to the problem landing page. |
|
ramp_frontend.forms
: forms used in the website¶
The ramp_frontend.forms
module defines the different forms used on the
website.
|
Login-in form. |
|
User profile form. |
|
User profile form. |
|
Code form. |
|
Submission name form. |
|
Submission uploading form. |
|
Form to update the parameters of an event. |
|
A form containing multiple checkboxes. |
|
The form allowing to select which model to view. |
|
Credit form. |
|
Form to ask for a new event. |
ramp_frontend.testing
: functionalities to test the frontend¶
The ramp_frontend.testing
module contains all functions used to
easily test the frontend.
|
Simulate a log-in from a user. |
|
Simulate a log-out. |
|
Context manager to log-in during the |
ramp_frontend.utils
: Utilities to ease sending email¶
The ramp_frontend.utils
provides utilities to ease sending email.
Create the body of an email using the user information. |
|
|
Send email using Flask Mail. |
RAMP utils¶
The RAMP utils package is made of utilities used for managing configuration, deploying RAMP events, and other utilities shared between the different RAMP packages.
Configuration utilities¶
|
Generate the configuration to deal with Flask. |
|
Generate the configuration to deploy RAMP. |
|
Generate the configuration for RAMP worker from a configuration file. |
|
Read and parse the configuration file for RAMP. |
Return the path a template database configuration file. |
|
Return the path a template RAMP configuration file. |
Datasets utilities¶
|
Fetch files from OSF storage. |
|
Deployment utilities¶
|
Deploy a RAMP event using a configuration file. |